Cohorten
App:
Cohorten Statistic:
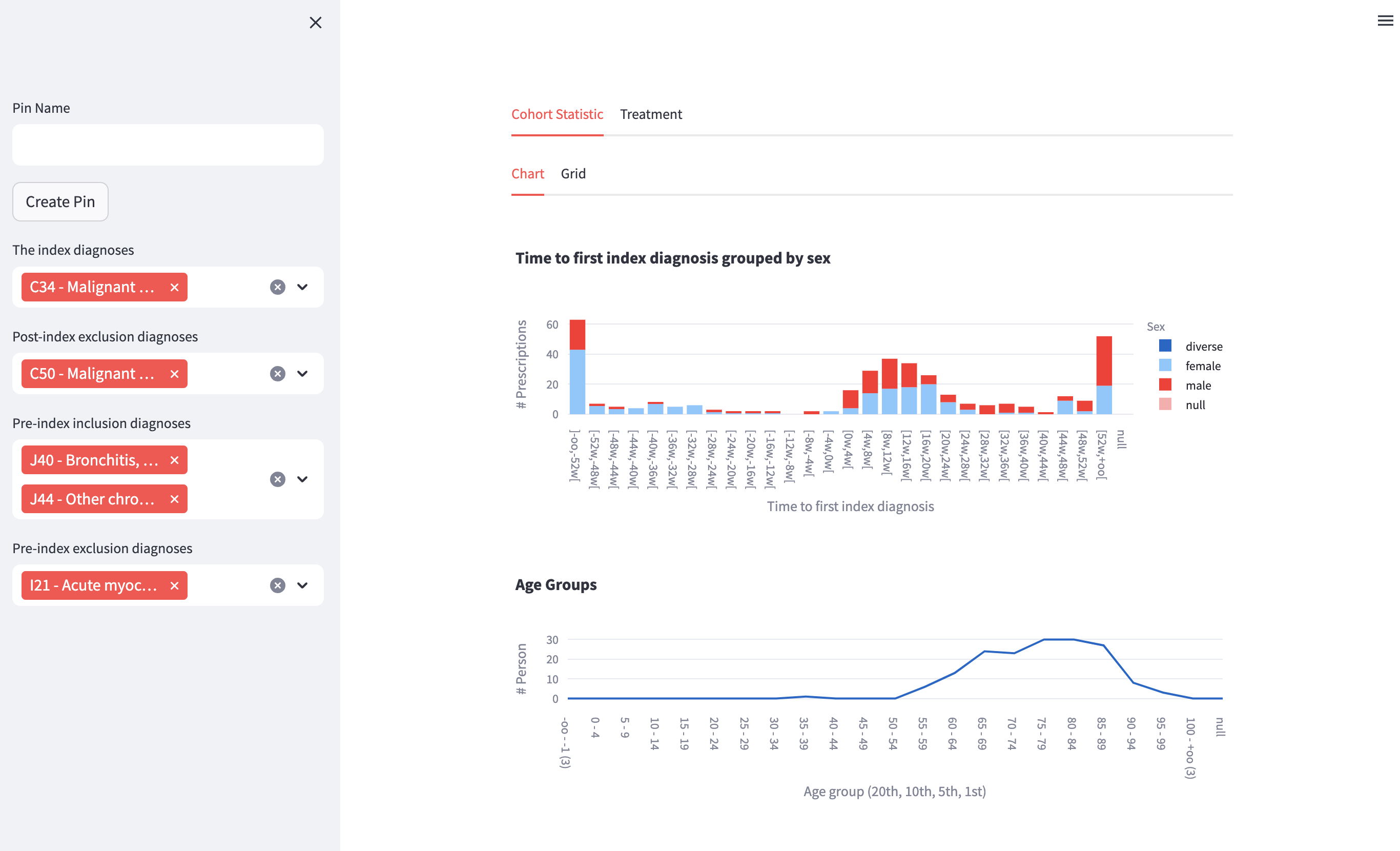
Streatment:
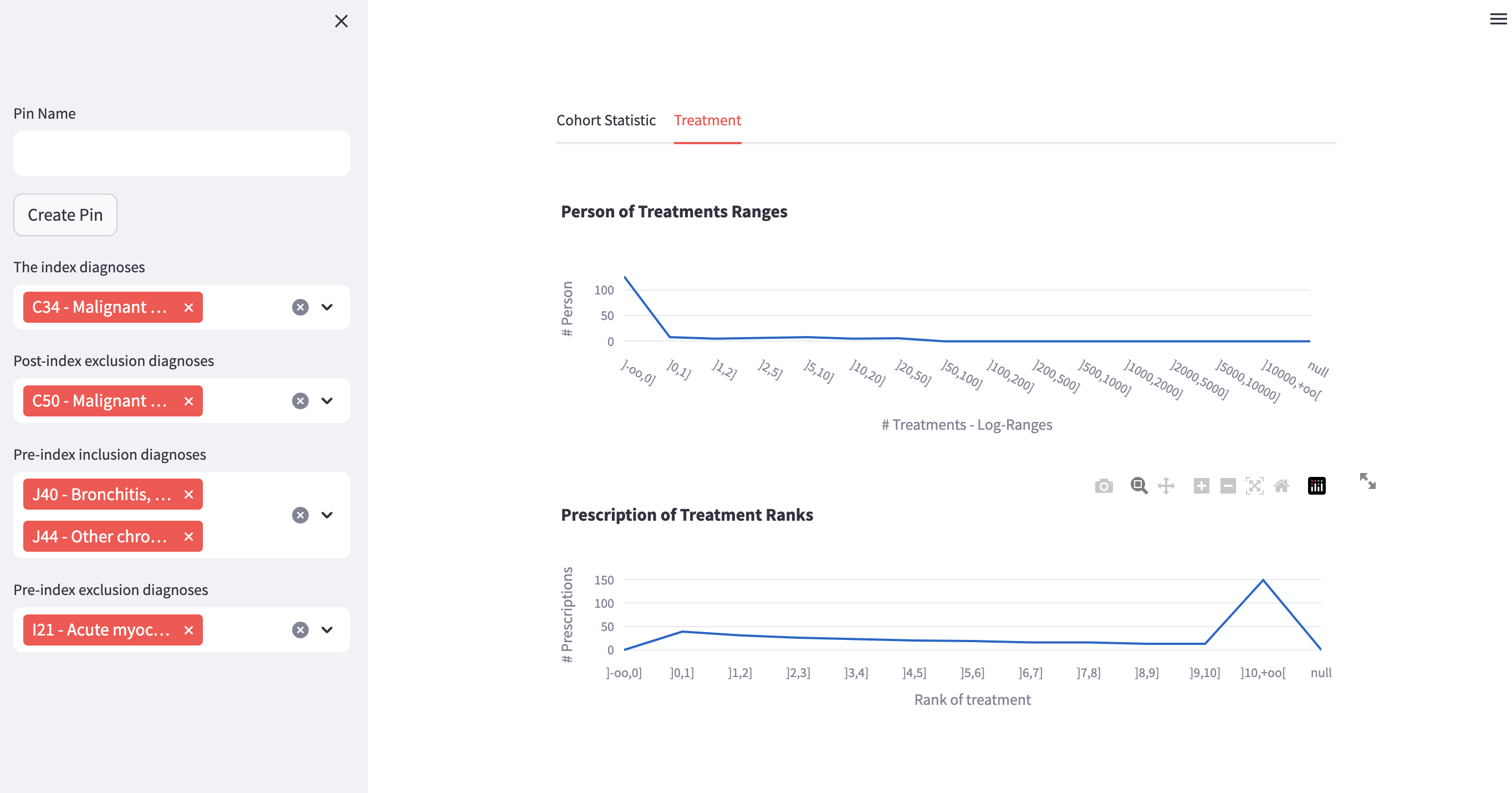
Code:
import streamlit as st
import xplain
import plotly.express as px
x = None
if "pins" not in st.session_state:
st.session_state.pins = []
st.title= "Cohorten Selection"
@st.cache_data
def get_xplainsession():
global x
print("perform start up")
x = xplain.Xsession()
x.startup('GFL_intern')
return x
@st.cache_data
def get_states():
global x
return x.perform({
"method": "getStatesListForLevel",
"object": "Diagnoses",
"dimension": "ICD",
"attribute": "ICD",
"levelNumber": 3,
"sort": True
}).get("statesOfLevel")
@st.cache_resource
def create_selection_sets():
global x
for selection_set in ["index_diagnoses",
"post_index_exclusion_diagnoses",
"pre_index_inclusion_diagnoses",
"pre_index_exclustion_giagnoses"]:
try:
x.run({
"method": "removeSelectionSet",
"name": selection_set})
x.run({
"method": "addSelectionSet",
"name": selection_set})
except:
print("{} exists ".format(selection_set))
@st.cache_resource
def create_relative_time_to_first_index_diagnoses():
'''
add Relative TimeDimension "Time to first index diagnosis" to Diagnoses using selection set index_diagnoses
'''
global x
try:
x.run({
"method": "removeDimensionIfExists",
"object":"Diagnoses",
"dimension":"Time to first index diagnosis"
})
print("remove if exists time to first diagnoses")
x.run({
"method": "addRelativeTimeDimensions",
"selectionSet": "index_diagnoses",
"referenceTimeDimension": {
"dimension": "Date of diagnosis",
"object": "Diagnoses"
},
"names": [
"Time to first index diagnosis"
],
"timeDimensions": [
{
"dimension": "Date of diagnosis",
"object": "Diagnoses"
}
],
"calendarBased": False,
"relativeTimeType": "TO_FIRST",
"baseObject": "Person",
"referenceEventSelections": [],
"attributeConfig": None,
"timeUnit": "MILLISECOND"})
print("Dimension Time to first index diagnosis added to Object Diagnoses")
x.run({
"searchStrategy": "BINARY_SEARCH",
"endpointClass": "java.lang.Long",
"method": "addTimeDurationAttribute",
"creationMode": "EQUIDISTANT",
"maxBaseUnits": 364,
"reduceUnits": True,
"baseUnit": "DAY",
"minBaseUnits": -364,
"upperBinBoundaryIncluded": False,
"attribute": "Time to first index diagnosis",
"levelNames": [
"Level 1 (4w)",
"Level 2 (1w)",
"Level 3 (1d)"
],
"dimensionTimeUnit": "MILLISECOND",
"dimension": "Time to first index diagnosis",
"equidistantCreationTimeUnitBinSizes": [
28,
7,
1
],
"object": "Diagnoses"})
print("equi dist attribute 'Time to first index diagnosis' added to Dimension ' Time to first index diagnosis'")
except:
pass
@st.cache_resource
def create_relative_time_to_first_index_prescription():
global x
try:
x.run({
"method": "removeDimensionIfExists",
"object":"Prescriptions",
"dimension":"Time to first index diagnosis"
})
print('remove if exists time to first prescription')
x.run({
"method": "addRelativeTimeDimensions",
"selectionSet": "index_diagnoses",
"referenceTimeDimension": {
"dimension": "Date of diagnosis",
"object": "Diagnoses"
},
"names": [
"Time to first index diagnosis"
],
"timeDimensions": [
{
"dimension": "Rx Order date",
"object": "Prescriptions"
}
],
"calendarBased": False,
"relativeTimeType": "TO_FIRST",
"baseObject": "Person",
"referenceEventSelections": [],
"attributeConfig": None,
"timeUnit": "MILLISECOND"})
print("added time dimesion prescription")
x.run({
"searchStrategy": "BINARY_SEARCH",
"endpointClass": "java.lang.Long",
"method": "addTimeDurationAttribute",
"creationMode": "EQUIDISTANT",
"maxBaseUnits": 364,
"reduceUnits": True,
"baseUnit": "DAY",
"minBaseUnits": -364,
"upperBinBoundaryIncluded": False,
"attribute": "Time to first index diagnosis",
"levelNames": [
"Level 1 (4w)",
"Level 2 (1w)",
"Level 3 (1d)"
],
"dimensionTimeUnit": "MILLISECOND",
"dimension": "Time to first index diagnosis",
"equidistantCreationTimeUnitBinSizes": [
28,
7,
1
],
"object": "Prescriptions"})
print("relt. time dim Time to first index diagnosis added to Prescription")
except:
pass
@st.cache_resource
def create_post_index_inclusion_dimension():
global x
x.run({
"method": "addAggregationDimension",
"selectionSet": "index_diagnoses",
"targetObject": "Person",
"selections": {
"selections": [
{
"attribute": {
"name": "Time to first index diagnosis",
"attribute": "Time to first index diagnosis",
"dimension": "Time to first index diagnosis",
"object": "Diagnoses"
},
"selectedStates": [
"[4w,8w[",
"[8w,12w["
]
}
],
"object": "Diagnoses"
},
"aggregation": {
"aggregationType": "COUNT",
"aggregationName": "# Diagnoses",
"complementMissingBranches": False,
"type": "COUNT",
"object": "Diagnoses"
},
"dimension": "Post-index inclusion diagnoses"
}
)
@st.cache_resource
def create_pre_index_inclusion_dimension():
global x
x.run({
"selectionSet": "pre_index_inclusion_diagnoses",
"targetObject": "Person",
"selections": {
"selections": [
{
"attribute": {
"name": "Time to first index diagnosis",
"attribute": "Time to first index diagnosis",
"dimension": "Time to first index diagnosis",
"object": "Diagnoses"
},
"selectedStates": [
"[-12w,-8w[",
"[-16w,-12w[",
"[-20w,-16w[",
"[-24w,-20w[",
"[-4w,0w[",
"[-8w,-4w["
]
}
],
"object": "Diagnoses"
},
"method": "addAggregationDimension",
"aggregation": {
"aggregationType": "COUNT",
"aggregationName": "# Diagnoses",
"complementMissingBranches": False,
"type": "COUNT",
"object": "Diagnoses"
},
"dimension": "Pre-index inclusion diagnoses"
})
@st.cache_resource
def create_pre_index_exclusion_dimension():
global x
x.run({
"method": "addAggregationDimension",
"dimension": "Pre-index exclusion diagnoses",
"selectionSet": "pre_index_exclustion_giagnoses",
"targetObject": "Person",
"selections": {
"selections": [
{
"attribute": {
"name": "Time to first index diagnosis",
"attribute": "Time to first index diagnosis",
"dimension": "Time to first index diagnosis",
"object": "Diagnoses"
},
"selectedStates": [
"[-12w,-8w[",
"[-16w,-12w[",
"[-20w,-16w[",
"[-24w,-20w[",
"[-4w,0w[",
"[-8w,-4w["
]
}
],
"object": "Diagnoses"
},
"aggregation": {
"aggregationType": "COUNT",
"aggregationName": "# Diagnoses",
"complementMissingBranches": False,
"type": "COUNT",
"object": "Diagnoses"
}
})
@st.cache_resource
def create_post_index_exclusion_dimension():
global x
x.run({
"selectionSet": "post_index_exclusion_diagnoses",
"targetObject": "Person",
"selections": {
"selections": [
{
"attribute": {
"name": "Time to first index diagnosis",
"attribute": "Time to first index diagnosis",
"dimension": "Time to first index diagnosis",
"object": "Diagnoses"
},
"selectedStates": [
"[0w,4w[",
"[4w,8w[",
"[8w,12w["
]
}
],
"object": "Diagnoses"
},
"method": "addAggregationDimension",
"aggregation": {
"aggregationType": "COUNT",
"aggregationName": "# Diagnoses",
"complementMissingBranches": False,
"type": "COUNT",
"object": "Diagnoses"
},
"dimension": "Post-index exclusion diagnoses"
})
@st.cache_resource
def create_treatment_dimension():
x.run({
"selectionSet": "globalSelections",
"targetObject": "Person",
"selections": [],
"method": "addAggregationDimension",
"aggregation": {
"aggregationType": "COUNT",
"aggregationName": "# Prescriptions",
"complementMissingBranches": False,
"type": "COUNT",
"object": "Prescriptions"
},
"dimension": "# Treatments"
})
@st.cache_resource
def build_rank_rx_order_date():
global x
x.run({
"selectionSet": "globalSelections",
"selections": [],
"dimensionClass": "RankDimension",
"method": "buildSortedSequence",
"rankDimensionName": "Rank of treatment",
"sortDimension": {
"dimension": "Rx Order date",
"object": "Prescriptions"
},
"type": "INT",
"reverse": False,
"baseObject": "Person",
"selectionSetDefiningRank": "globalSelections"
})
@st.cache_resource
def prop_selection_upw(b_upwards):
global x
x.run({
"method": "setPropagateSelectionsUpward",
"propagateSelectionsUpward": False
})
@st.cache_resource
def set_global_selections():
global x
x.run({
"method": "select",
"selectionSet": "globalSelections",
"selections": [
{
"attribute": {
"name": "ICD",
"attribute": "ICD",
"dimension": "ICD",
"object": "Diagnoses"
},
"selectedStates": [
"C33 - Malignant neoplasm of trachea",
"C34 - Malignant neoplasm of bronchus and lung",
"C44 - Other malignant neoplasms of skin",
"C50 - Malignant neoplasm of breast",
"C61 - Malignant neoplasm of prostate",
"I21 - Acute myocardial infarction",
"I64 - Stroke, not specified as haemorrhage or infarction",
"J20-J22 - Other acute lower respiratory infections",
"J40-J47 - Chronic lower respiratory diseases"
]
},
{
"attribute": {
"name": "Post-index exclusion diagnoses - Log-Ranges",
"attribute": "Post-index exclusion diagnoses - Log-Ranges",
"dimension": "Post-index exclusion diagnoses",
"object": "Person"
},
"selectedStates": [
"]-oo,0]"
]
},
{
"attribute": {
"name": "Post-index inclusion diagnoses - Log-Ranges",
"attribute": "Post-index inclusion diagnoses - Log-Ranges",
"dimension": "Post-index inclusion diagnoses",
"object": "Person"
},
"selectedStates": [
"]0,1]",
"]1,2]",
"]10,20]",
"]100,200]",
"]1000,2000]",
"]10000,+oo[",
"]2,5]",
"]20,50]",
"]200,500]",
"]2000,5000]",
"]5,10]",
"]50,100]",
"]500,1000]",
"]5000,10000]"
]
},
{
"attribute": {
"name": "Pre-index exclusion diagnoses - Log-Ranges",
"attribute": "Pre-index exclusion diagnoses - Log-Ranges",
"dimension": "Pre-index exclusion diagnoses",
"object": "Person"
},
"selectedStates": [
"]-oo,0]"
]
},
{
"attribute": {
"name": "Pre-index inclusion diagnoses - Log-Ranges",
"attribute": "Pre-index inclusion diagnoses - Log-Ranges",
"dimension": "Pre-index inclusion diagnoses",
"object": "Person"
},
"selectedStates": [
"]0,1]",
"]1,2]",
"]10,20]",
"]100,200]",
"]1000,2000]",
"]10000,+oo[",
"]2,5]",
"]20,50]",
"]200,500]",
"]2000,5000]",
"]5,10]",
"]50,100]",
"]500,1000]",
"]5000,10000]"
]
},
{
"attribute": {
"name": "MP PCN ATC",
"attribute": "MP PCN ATC",
"dimension": "Rx PCN",
"object": "Prescriptions"
},
"selectedStates": [
"L - Antineoplastic and immunomodulating agents"
]
}
]
})
@st.cache_resource
def open_prec_over_time_2_first_index_and_gender():
global x
x.run({"method":"deleteRequest", "requestName": "Time_to_first_index_diagnosis"})
x.run({
"method": "openQuery" ,
"request": {
"requestName": "Time_to_first_index_diagnosis",
"groupBys": [
{
"groupByName": "group by Time to first index diagnosis.Sex",
"subGroupings": [
{
"groupByName": "GROUP BY Time to first index diagnosis",
"groupByLevelNumber": 1,
"groupByStatesFormHierarchyWithRoot": "All durations",
"includeUpperLevels": False,
"attribute": {
"name": "Time to first index diagnosis",
"attribute": "Time to first index diagnosis",
"dimension": "Time to first index diagnosis",
"object": "Prescriptions"
}
},
{
"groupByName": "group by Prescriptions",
"groupByStatesFormHierarchyWithRoot": "Sex",
"includeUpperLevels": False,
"attribute": {
"name": "Sex",
"attribute": "Sex",
"dimension": "Sex",
"object": "Person"
}
}
]
}
],
"externalSelections": "globalSelections",
"aggregations": [
{
"aggregationType": "COUNT",
"aggregationName": "# Prescriptions",
"complementMissingBranches": False,
"type": "COUNT",
"object": "Prescriptions"
}
]
}})
print("open query called")
@st.cache_resource
def open_age_group():
global x
x.run({"method":"deleteRequest", "requestName": "Agegroup"})
x.run({
"method": "openQuery",
"request": {
"requestName": "Agegroup",
"groupBys": [
{
"groupByName": "GROUP BY Age group (20th, 10th, 5th, 1st)",
"groupByLevelNumber": 3,
"groupByStatesFormHierarchyWithRoot": "-oo - +oo",
"includeUpperLevels": True,
"attribute": {
"name": "Age group (20th, 10th, 5th, 1st)",
"attribute": "Age group (20th, 10th, 5th, 1st)",
"dimension": "Date of birth",
"object": "Person"
}
}
],
"externalSelections": "globalSelections",
"aggregations": [
{
"aggregationType": "COUNT",
"aggregationName": "# Person",
"complementMissingBranches": False,
"type": "COUNT",
"object": "Person"
}
]
}
})
@st.cache_resource
def open_treatment_log_range():
global x
x.run({"method":"deleteRequest", "requestName": "openTreatments-Log-Ranges"})
x.run({
"request": {
"requestName": "openTreatments-Log-Ranges",
"groupBys": [
{
"groupByName": "GROUP BY # Treatments - Log-Ranges",
"groupByLevelNumber": 1,
"groupByStatesFormHierarchyWithRoot": "]-oo,+oo[",
"includeUpperLevels": True,
"attribute": {
"name": "# Treatments - Log-Ranges",
"attribute": "# Treatments - Log-Ranges",
"dimension": "# Treatments",
"object": "Person"
}
}
],
"externalSelections": "globalSelections",
"aggregations": [
{
"aggregationType": "COUNT",
"aggregationName": "# Person",
"complementMissingBranches": False,
"type": "COUNT",
"object": "Person"
}
]
},
"method": "openQuery"
})
@st.cache_resource
def open_rank_treatment():
global x
x.run({"method":"deleteRequest", "requestName": "Rank of treatment"})
x.run({
"method": "openQuery",
"request": {
"requestName": "Rank of treatment",
"groupBys": [
{
"groupByName": "GROUP BY Rank of treatment",
"groupByLevelNumber": 1,
"groupByStatesFormHierarchyWithRoot": "]-oo,+oo[",
"includeUpperLevels": False,
"attribute": {
"name": "Rank of treatment",
"attribute": "Rank of treatment",
"dimension": "Rank of treatment",
"object": "Prescriptions"
}
}
],
"externalSelections": "globalSelections",
"aggregations": [
{
"aggregationType": "COUNT",
"aggregationName": "# Prescriptions",
"complementMissingBranches": False,
"type": "COUNT",
"object": "Prescriptions"
}
]
}
})
x = get_xplainsession()
def create_pin(pin_name):
if pin_name is not None:
st.session_state.pins.append(pin_name)
icd_states = get_states()
create_selection_sets()
create_relative_time_to_first_index_diagnoses()
create_relative_time_to_first_index_prescription()
print(x.show_tree())
create_pre_index_inclusion_dimension()
create_pre_index_exclusion_dimension()
create_post_index_inclusion_dimension()
create_post_index_exclusion_dimension()
create_treatment_dimension()
build_rank_rx_order_date()
prop_selection_upw(False)
set_global_selections()
open_prec_over_time_2_first_index_and_gender()
open_age_group()
open_treatment_log_range()
open_rank_treatment()
# Using "with" notation
with st.sidebar:
pin_name = st.text_input('Pin Name')
if st.button("Create Pin"):
create_pin(pin_name)
index_diag_selections = st.multiselect(
'The index diagnoses',
icd_states,
['C34 - Malignant neoplasm of bronchus and lung']
)
post_index_diag_exclusion_selections = st.multiselect(
'Post-index exclusion diagnoses',
icd_states,
['C50 - Malignant neoplasm of breast']
)
pre_index_inclu_diag_selections = st.multiselect(
'Pre-index inclusion diagnoses',
icd_states,
['J40 - Bronchitis, not specified as acute or chronic',
'J44 - Other chronic obstructive pulmonary disease']
)
pre_index_exclu_diag_selections = st.multiselect(
'Pre-index exclusion diagnoses',
icd_states,
['I21 - Acute myocardial infarction']
)
for checkbox in st.session_state.pins:
st.checkbox(checkbox)
if "index_diag_selections" not in st.session_state:
st.session_state.index_diag_selections = []
if index_diag_selections != st.session_state.index_diag_selections:
print("index_diag_selections: " + str(index_diag_selections))
x.run({
"method": "select",
"selectionSet": "index_diagnoses",
"selections": [
{
"attribute": {
"name": "ICD",
"attribute": "ICD",
"dimension": "ICD",
"object": "Diagnoses"
},
"selectedStates": index_diag_selections
}
]
})
st.session_state.index_diag_selections = index_diag_selections
if "post_index_diag_exclusion_selections" not in st.session_state:
st.session_state.post_index_diag_exclusion_selections = []
if post_index_diag_exclusion_selections != st.session_state.post_index_diag_exclusion_selections :
print("post_index_diag_exclusion_selections: " + str(post_index_diag_exclusion_selections))
x.run({
"method": "select",
"selectionSet": "post_index_exclusion_diagnoses",
"selections": [
{
"attribute": {
"name": "ICD",
"attribute": "ICD",
"dimension": "ICD",
"object": "Diagnoses"
},
"selectedStates": post_index_diag_exclusion_selections
}
]
})
st.session_state.post_index_diag_exclusion_selections = post_index_diag_exclusion_selections
if "pre_index_inclu_diag_selections" not in st.session_state:
st.session_state.pre_index_inclu_diag_selections = []
if pre_index_inclu_diag_selections != st.session_state.pre_index_inclu_diag_selections:
print("pre_index_inclu_diag_selections: " + str(pre_index_inclu_diag_selections))
x.run({
"method": "select",
"selectionSet": "pre_index_inclusion_diagnoses",
"selections": [
{
"attribute": {
"name": "ICD",
"attribute": "ICD",
"dimension": "ICD",
"object": "Diagnoses"
},
"selectedStates": pre_index_inclu_diag_selections
}
]
})
st.session_state.pre_index_inclu_diag_selections = pre_index_inclu_diag_selections
if "pre_index_exclu_diag_selections" not in st.session_state:
st.session_state.pre_index_exclu_diag_selections = []
if pre_index_exclu_diag_selections != st.session_state.pre_index_exclu_diag_selections :
print("pre_index_exclu_diag_selections: " + str(pre_index_exclu_diag_selections))
x.run({
"method": "select",
"selectionSet": "pre_index_exclustion_giagnoses",
"selections": [
{
"attribute": {
"name": "ICD",
"attribute": "ICD",
"dimension": "ICD",
"object": "Diagnoses"
},
"selectedStates": pre_index_exclu_diag_selections
}
]
})
st.session_state.pre_index_exclu_diag_selections = pre_index_exclu_diag_selections
tab1, tab2 = st.tabs(["Cohort Statistic", "Treatment"])
with tab1:
df_time_to_fist_index= x.get_result("Time_to_first_index_diagnosis")
df_age_group = x.get_result('Agegroup')
tab1_chart, tab1_grid = st.tabs(["Chart", "Grid"])
with tab1_chart:
#st.button("Create Pin")
fig = px.bar(
df_time_to_fist_index,
x='Time to first index diagnosis',
y='# Prescriptions',
color="Sex",
height=300,
title="Time to first index diagnosis grouped by sex" )
fig_age = px.line(df_age_group,
x="Age group (20th, 10th, 5th, 1st)",
y="# Person",
height=250,
title="Age Groups")
st.plotly_chart(fig, theme="streamlit", use_container_width=False)
st.plotly_chart(fig_age, theme="streamlit", use_container_width=False)
with tab1_grid:
st.write(df_time_to_fist_index)
st.write(df_age_group)
with tab2:
df_treatment = x.get_result('openTreatments-Log-Ranges')
#st.write(df_treatment)
fig_treatment = px.line(df_treatment,
x="# Treatments - Log-Ranges",
y="# Person",
height=250,
title="Person of Treatments Ranges")
st.plotly_chart(fig_treatment)
df_rank = x.get_result('Rank of treatment')
fig_rank = px.line(df_rank,
x="Rank of treatment",
y="# Prescriptions",
height=250,
title="Prescription of Treatment Ranks")
st.plotly_chart(fig_rank)
#st.write(df_rank)
Packages that should be entered in the pip install field: xplain, plotly, streamlit